Often during the development of a Laravel application, there are cases where it is necessary to implement the debugged code on a live server. It takes a long time to transfer all the code (including packages and modules) even at a high transfer rate. The solution is to update only the latest, changed code files.
In the case when the (vendor) packages are unchanged, and we need to update the files of our own application, we can use the incremental file transfer using the git versioning system.
A prerequisite is the regular creation of versions of the code using git, to generate a package of changed files.
We present an example of using this approach in an Ubuntu environment (Windows 11, WSL2, PhpStorm 2022.2).
The basis of the solution is a bash file using git commands:
#!/usr/bin/env bash # script for creating zip file containing all changed files after `revision` commit up to HEAD commit # resulting zip file `package_<datetime>_<revision_leading_8_chars>` is stored in ` deploy` folder # revision number of `previous` commit to revision which have to be included into output zip file revision=eaae368c827a3c78f10787cb90f72a2381ee791e now="$(date +'%g%m%d_%H%M')__" short=${revision:0:8} git archive --output=./deploy/package_$now$short.zip HEAD $(git diff --name-only $revision..HEAD --diff-filter=d) printf "%s\n" $(git diff --name-only $revision..HEAD --diff-filter=d)
The git archive
command creates a zip file with all changed files between the older revision (specified by the revision number) and the HEAD version.
Copying the revision number in Git(PhpStorm):
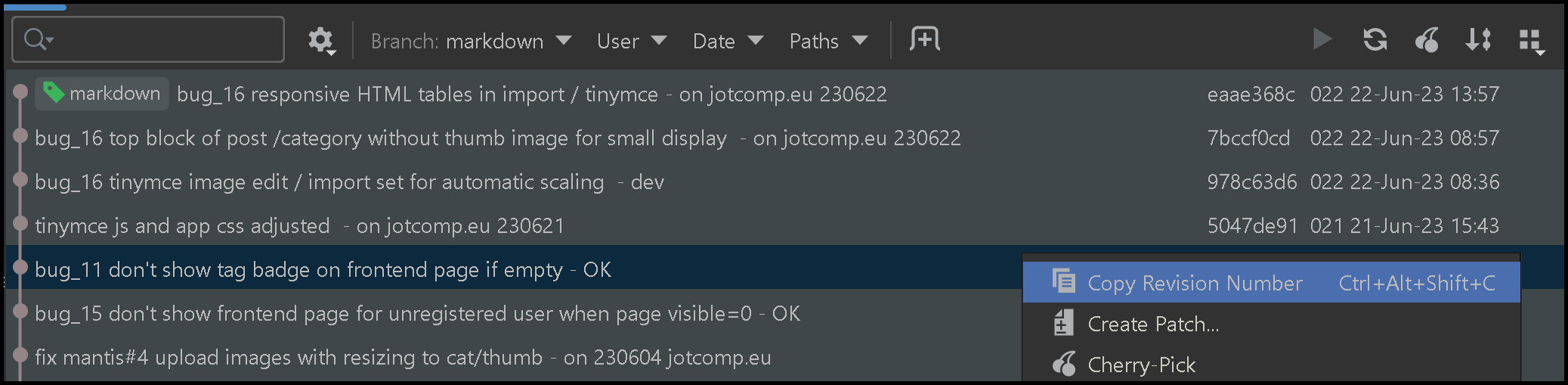
The files in the zip package are stored together with the relative paths, so they can be directly mass copied to the live server.